Lý thuyết cần xem qua
#1. Ag-grid là gì?
Ag-grid là một thư viện js được dùng hỗ trợ việc hiển thị dữ liệu dưới dạng bảng biểu trong javascript và các framework của nó.
Nó hỗ trợ từ những chức năng cơ bản đến phức tạp mà một bảng biểu cần phải có như:
- Tạo cột, điều chỉnh chiều rộng, chiều cao, wrap text
- Sort, filter dữ liệu, kéo thả vị trí các cột trên UI, đặt cố định một số cột
- Nhập liệu trực tiếp, format dữ liệu…
Hoặc bạn có thể tuỳ ý thêm các tính năng khác cho grid như:
- Validate dữ liệu nhập
- Tạo custom input gắn liền với từng cells
- Chèn công thức tính toán như trên excel
ag-grid có bản Community (miễn phí) và Enterprise (mất phí). Bản Enterprise có thêm một số các tính năng hỗ trợ cao cấp hơn (như xuất file excel, thay vì chỉ được xuất csv ở bản miễn phí). Tuy nhiên để học và tìm hiểu hoặc làm project nhỏ thì bản Community đã quá đầy đủ các chức năng cần thiết rồi.
#2. @ViewChild là gì?
Trong ví dụ dưới đây ta sẽ có làm việc với @ViewChild, vậy @ViewChild là gì?
Angular @ViewChild
decorator là 1 trong những decorator đầu tiên mà chúng ta sẽ học khi tìm hiểu về Angular.
Khi chúng ta muốn truy cập vào các child component, directive hay DOM element từ parent component, ViewChild decorator sẽ giúp chúng ta điều này.
ViewChild trả về phần tử đầu tiền mà chúng ta muốn truy vấn. Trong trường hợp chúng ta muốn truy vấn tới nhiều phần từ con, chúng ta có thể dùng ViewChildren
thay thế.
Để hiểu rõ thêm về @ViewChild mời bạn xem tiếp tại đây
Bắt đầu với AG Grid Angular
Để thực hành và có được kết quả như bài viết này Chúng ta cần phải cài đặt môi trường Dev trước khi bắt đầu.
#1. Khởi tạo dự án
$ cd temp/ $ ng new my-app --style scss --routing false $ cd my-app/ $ code .
Khởi chạy server
$ ng serve #hoặc $ npm start
#2. Đưa AG Grid vào dự án
#1. Thêm package Ag Grid
vào package.json
$ npm install --save ag-grid-community ag-grid-angular $ npm install
#2. Thêm AG Grid Angular module
vào app module
– src/app/app.module.ts
import { AgGridModule } from 'ag-grid-angular'; imports: [ AgGridModule.withComponents([]) ]
#3. Đưa style
của ag-grid vào ứng dụng– src/styles.scss
@import "../node_modules/ag-grid-community/dist/styles/ag-grid.css"; @import "../node_modules/ag-grid-community/dist/styles/ag-theme-balham.css";
#4. Tạo Column Definitions cơ bản– src/app/app.component.ts
columnDefs = [ { field: 'make' }, { field: 'model' }, { field: 'price'} ]; //Hard-code dữ liệu hiển thị cho grid rowData = [ { make: 'Toyota', model: 'Celica', price: 35000 }, { make: 'Ford', model: 'Mondeo', price: 32000 }, { make: 'Porsche', model: 'Boxter', price: 72000 } ];
#5. Đưa ag-grid vào template– app/app.component.html
<ag-grid-angular style="width: 500px; height: 500px;" class="ag-theme-balham" [rowData]="rowData" [columnDefs]="columnDefs" ></ag-grid-angular>
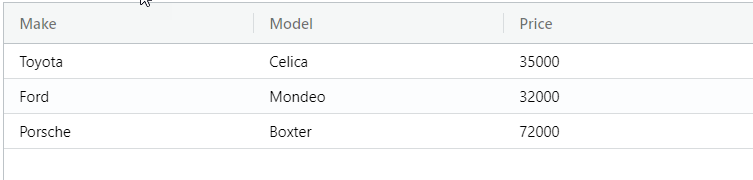
#3. Cho phép grid có thể sorting
src/app/app.component.ts
//Cài đặt lại column definitions columnDefs = [ { field: 'make', sortable: true }, { field: 'model', sortable: true }, { field: 'price', sortable: true } ];
#4. Cho phép grid có thể filtering
src/app/app.component.ts
//Cài đặt lại column definitions columnDefs = [ { field: 'make', sortable: true, filter: true }, { field: 'model', sortable: true, filter: true }, { field: 'price', sortable: true, filter: true } ];
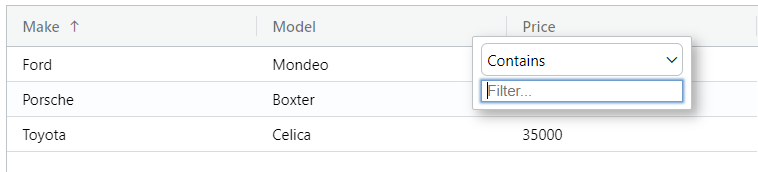
#5. Hiển thị dữ liệu tải từ remote
#1. Thêm HttpClient Angular Module
vào app module
– src/app/app.module.ts
import { HttpClientModule } from '@angular/common/http'; imports: [ HttpClientModule
#2. Code tải dữ liệu từ remote– src/app/app.component.ts
import { Component, OnInit } from '@angular/core'; import { HttpClient } from '@angular/common/http'; import {Observable} from 'rxjs'; export class AppComponent implements OnInit { rowData: Observable<any[]>; constructor(private http: HttpClient) { } ngOnInit(): void { this.rowData = this.http.get<any[]>( 'https://www.ag-grid.com/example-assets/small-row-data.json' ); }
#3. Cài đặt template– app/app.component.html
<ag-grid-angular style="width: 500px; height: 500px;" class="ag-theme-balham" [rowData]="rowData | async" [columnDefs]="columnDefs" ></ag-grid-angular>
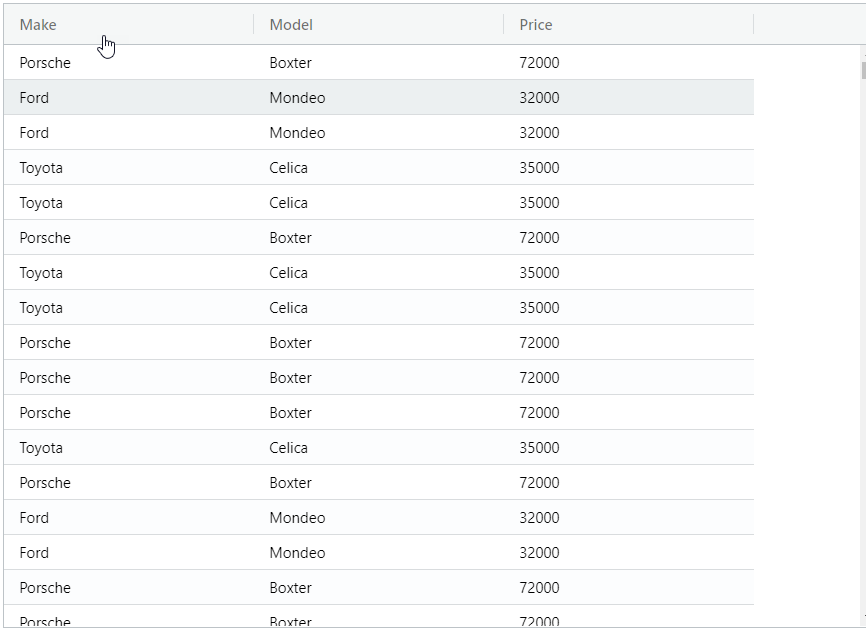
#6. Tạo column có checkbox
#1. Cho column có checkbox, cài đặt columnDefs– src/app/app.component.ts
columnDefs = [ {field: 'make', sortable: true, filter: true, checkboxSelection: true}, {field: 'model', sortable: true, filter: true}, {field: 'price', sortable: true, filter: true} ];
#2. Cho grid chọn được nhiều dòng, cài đặt template– app/app.component.html
<ag-grid-angular style="width: 500px; height: 500px;" class="ag-theme-balham" [rowData]="rowData | async" [columnDefs]="columnDefs" rowSelection="multiple" ></ag-grid-angular>
#7. Truy cập dữ liệu trên grid
#1. Tạo button với event click để hiển thị tin nhắn là dữ liệu của các dòng được chọn, thêm vào template– app/app.component.html
<button (click)="getSelectedRows()">Get Selected Rows</button>
#2. Tạo instance của grid
a) Cài đặt template– app/app.component.html
<ag-grid-angular #agGrid
b) Code hiện thị tin nhắn, sử dụng @ViewChild tạo instance– src/app/app.component.ts
import {Component, OnInit, ViewChild} from '@angular/core'; export class AppComponent implements OnInit { //Tạo instance agGrid component @ViewChild('agGrid') agGrid: AgGridAngular; } //Xử lý sự kiện click- đọc ra các dòng đã chọn getSelectedRows(): void { const selectedNodes = this.agGrid.api.getSelectedNodes(); const selectedData = selectedNodes.map(node => node.data); const selectedDataStringPresentation = selectedData .map(node => `${node.make} ${node.model}`) .join(', '); alert(`Selected nodes: ${selectedDataStringPresentation}`); }
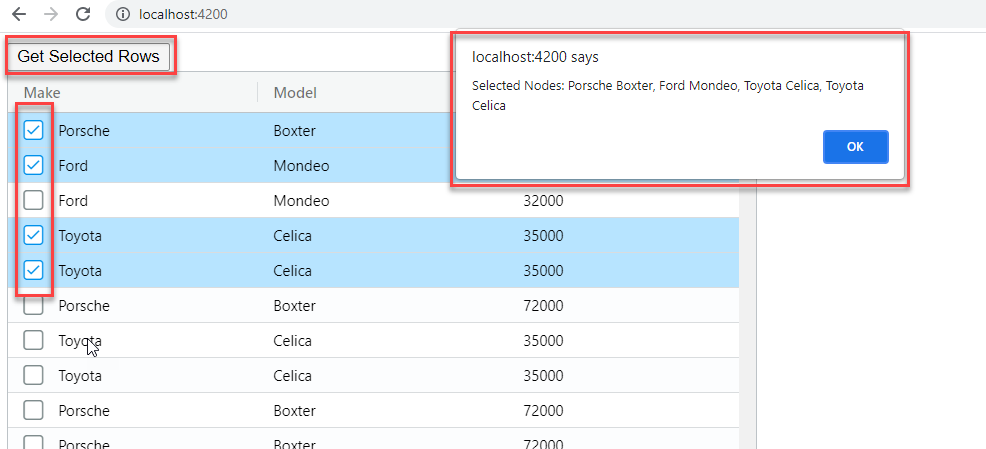
#8. Tạo Group Column
“Grouping is a feature exclusive to AG Grid Enterprise. You are free to trial AG Grid Enterprise to see what you think. You only need to get in touch if you want to start using AG Grid Enterprise in a project intended for production”
#1. Thêm package AG Grid Enterprise
vào package.json
$ npm install --save ag-grid-enterprise
#2. Thêm import
vào app module
– app.module.ts
import 'ag-grid-enterprise';
“If everything is ok, you should see a message in the console that tells you there is no enterprise license key. You can ignore the message as we are trialing”
#3. Cài đặt Group Column Definitions và tùy chỉnh lại columnDefs– src/app/app.component.ts
//Cài đặt áp dụng cho tất cả các column defaultColDef = { sortable: true, filter: true }; //Cài đặt Column columnDefs = [ { field: 'make', rowGroup: true }, { field: 'price' } ]; //Cài đặt Group Column autoGroupColumnDef = { headerName: 'Model', field: 'model', cellRenderer: 'agGroupCellRenderer', cellRendererParams: { checkbox: true } };
#4. Cho grid có Group Column, cài đặt template– app/app.component.html
<ag-grid-angular style="width: 500px; height: 500px;" class="ag-theme-balham" [defaultColDef]="defaultColDef" [autoGroupColumnDef]="autoGroupColumnDef" [rowData]="rowData | async" [columnDefs]="columnDefs" rowSelection="multiple" ></ag-grid-angular>
#5. Cho Group Column check chọn được children, cài đặt template– app/app.component.html
<ag-grid-angular style="width: 500px; height: 500px;" class="ag-theme-balham" [defaultColDef]="defaultColDef" [autoGroupColumnDef]="autoGroupColumnDef" [groupSelectsChildren]=true [rowData]="rowData | async" [columnDefs]="columnDefs" rowSelection="multiple" ></ag-grid-angular>
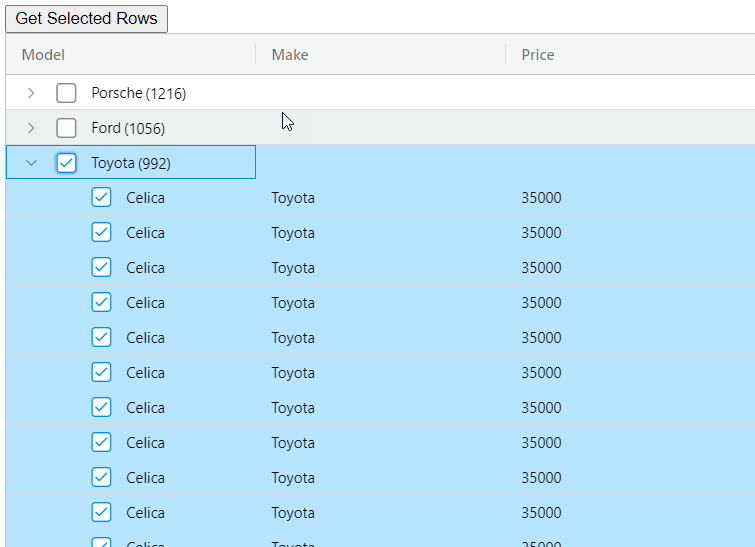
Tham khảo
Theo Angular Grid: Get Started with AG Grid
Tải source code về tại đây