Giới thiệu
Chúng ta có thể thêm hoặc bớt cột bằng cách cập nhật danh sách Column Definitions.
Khi các column mới được thiết lập, grid sẽ so sánh với các column hiện tại và tìm ra column nào cũ (sẽ bị loại bỏ), mới (column mới được tạo) hoặc được giữ lại.
Lý thuyết cần xem qua
Client-side Row Model là gì?
Một trong những Row Model dễ sử dụng nhất là Client Side Row Model, Row model này sẽ lấy tất cả dữ liệu để hiển thị ra view, kèm theo đó là các tính năng rất hữu dụng: Filtering, Sorting, Grouping, Aggregation, Pivoting.
Client Side Row Model là row model mặc định trong Ag-grid và được sử dụng hầu hết trong các trường hợp và chịu trách nhiệm hiển thị các rows trong grid. Cấu trúc dữ liệu của nó khá phức tạp, biểu diễn dữ liệu dưới nhiều trạng thái (state) khác nhau.
Để hiểu rõ thêm về Client-side Row Model này, mời bạn xem tiếp tại đây
Xét một ví dụ cụ thể
#1. Trang “Include Medal Columns”
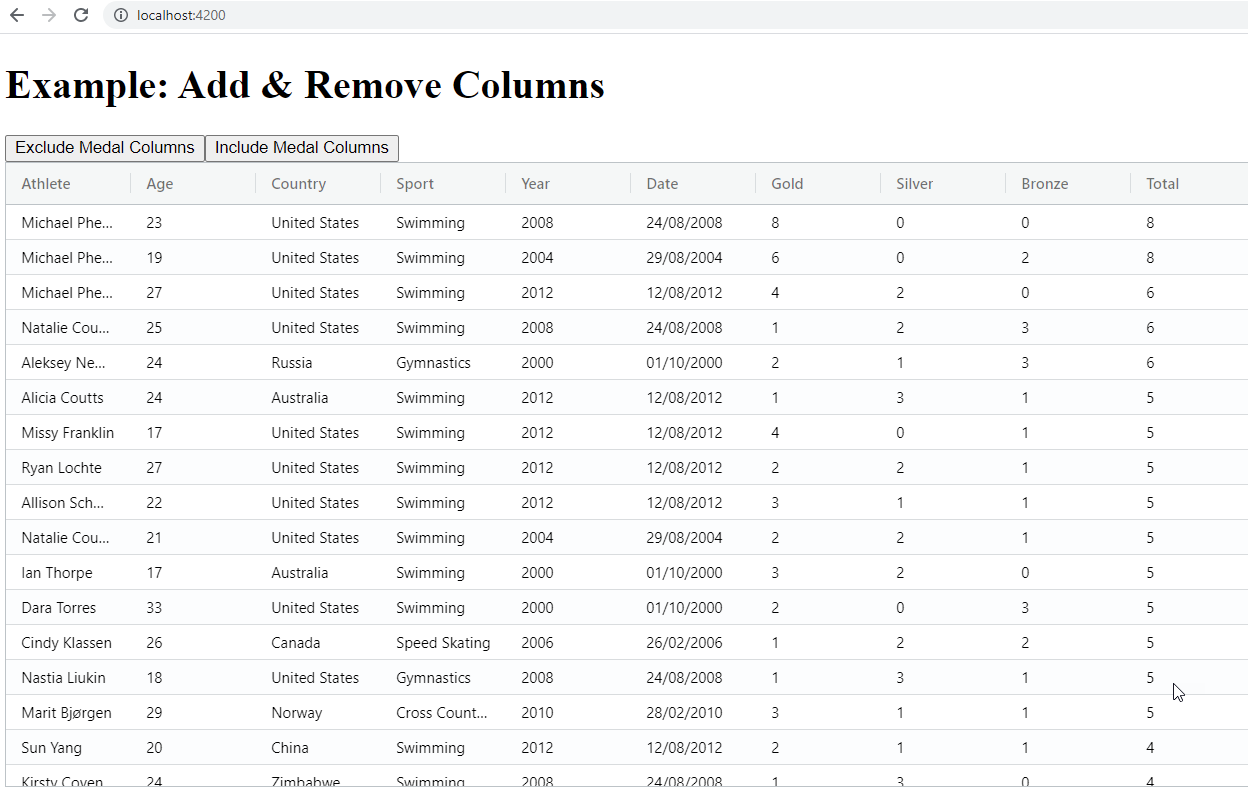
#2. Trang “Exclude Medal Columns”
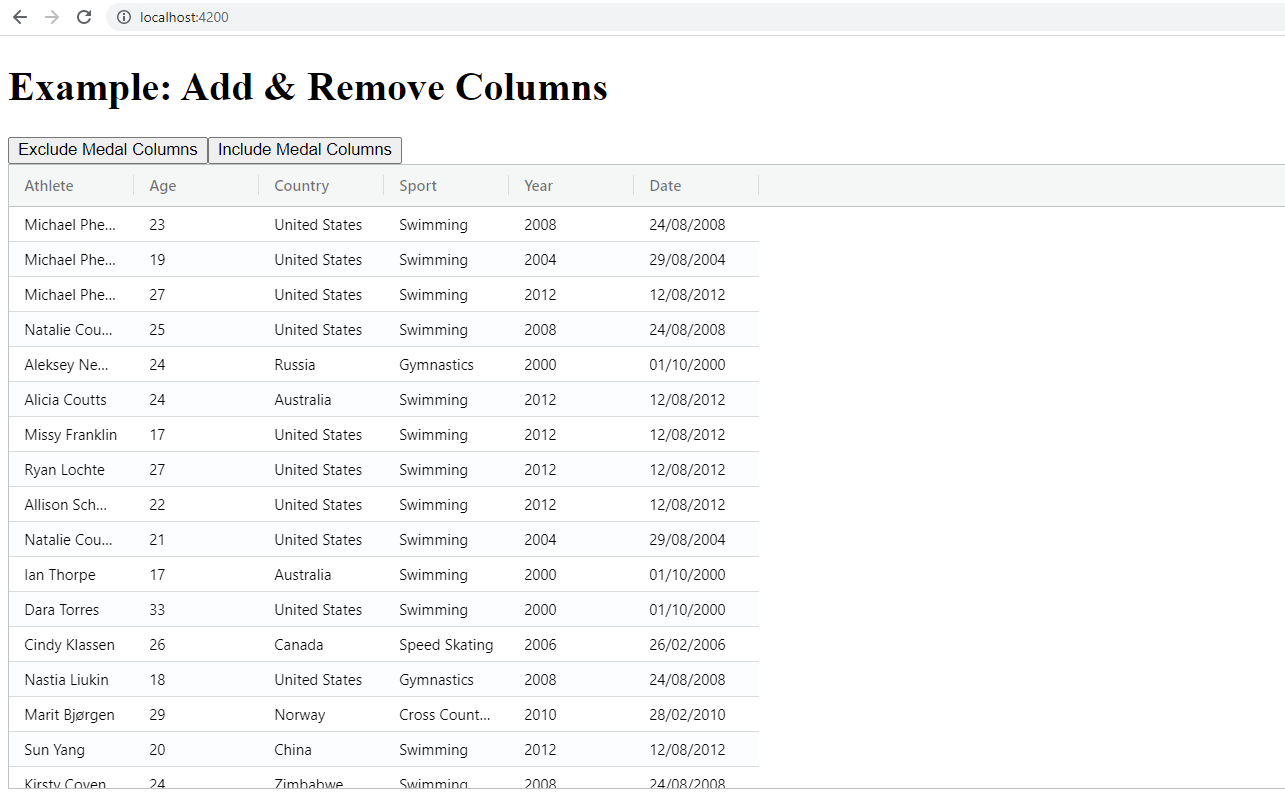
#1. Khởi tạo và cài đặt nhanh grid
Xem hướng dẫn khởi tạo và cài đặt nhanh grid cơ bản cho dự án tại đây
#2. Cài đặt thư viện cần thiết
$ npm install --save @ag-grid-community/client-side-row-model
#3. Code cho app component
Src\app\app.component.ts
import { Component } from '@angular/core'; import { HttpClient } from '@angular/common/http'; import { ClientSideRowModelModule } from '@ag-grid-community/client-side-row-model'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.scss'], }) export class AppComponent { //#1.Tạo objects cho việc truy cập tới grid private gridApi: any; private gridColumnApi: any; rowData: any; //Client-side Row Model public modules: any = [ClientSideRowModelModule]; //#2.Cài đặt mặc định áp dụng cho tất cả các column defaultColDef = { initialWidth: 100, sortable: true, resizable: true, }; //Cài đặt constructor constructor(private http: HttpClient) {} //#3.1 Xử lý sự kiện ngay khi grid được khởi tạo onGridReady(params: any) { //Khởi tạo object truy cập tới grid this.gridApi = params.api; this.gridColumnApi = params.columnApi; //Tải dữ liệu, hiện thị lên grid this.http .get('https://www.ag-grid.com/example-assets/olympic-winners.json') .subscribe((data) => { this.onBtIncludeMedalColumns(); this.rowData = data; }); } //#3.2.Xử lý sự kiện loại bỏ columns onBtExcludeMedalColumns() { this.gridApi.setColumnDefs(colDefsMedalsExcluded); } //#3.3.Xử lý sự kiện thêm columns onBtIncludeMedalColumns() { this.gridApi.setColumnDefs(colDefsMedalsIncluded); } } //#4.Cài đặt columns cho "Include Medal Columns" var colDefsMedalsIncluded = [ { field: 'athlete' }, { field: 'age' }, { field: 'country' }, { field: 'sport' }, { field: 'year' }, { field: 'date' }, { field: 'gold' }, { field: 'silver' }, { field: 'bronze' }, { field: 'total' }, ]; //#5.Cài đặt columns cho "Exclude Medal Columns" var colDefsMedalsExcluded = [ { field: 'athlete' }, { field: 'age' }, { field: 'country' }, { field: 'sport' }, { field: 'year' }, { field: 'date' }, ];
Khi các column mới được thiết lập, grid sẽ so sánh với các column hiện tại và tìm ra column nào cũ (sẽ bị loại bỏ), mới (column mới được tạo) hoặc được giữ lại.
#4. Dữ liệu grid hiện thị
Dưới đây là một phần dữ liệu được hiện thị trên grid, để xem đầy đủ xem tại đây
[ { "athlete": "Michael Phelps", "age": 23, "country": "United States", "year": 2008, "date": "24/08/2008", "sport": "Swimming", "gold": 8, "silver": 0, "bronze": 0, "total": 8 }, { "athlete": "Michael Phelps", "age": 19, "country": "United States", "year": 2004, "date": "29/08/2004", "sport": "Swimming", "gold": 6, "silver": 0, "bronze": 2, "total": 8 }, ]
#5. Code cho app template
Src\app\app.component.html
<div class="test-container"> <h3> Example: Add & Remove Columns</h3> <div class="test-header"> <button (click)="onBtExcludeMedalColumns()">Exclude Medal Columns</button> <button (click)="onBtIncludeMedalColumns()">Include Medal Columns</button> </div> <ag-grid-angular #agGrid style="width: 100%; height: 500px" id="myGrid" class="ag-theme-balham" [modules]="modules" [defaultColDef]="defaultColDef" [rowData]="rowData" (gridReady)="onGridReady($event)" ></ag-grid-angular> </div>
vì [ClientSideRowModelModule]
là mặc định trong Ag-grid nên ta có thể bỏ cài đặt [modules]="modules"
mà không ảnh hưởng đến kết quả của ví dụ.
#6. Kết quả
Tải lại trang, ta được kết quả như mong đợi… Thế là xong!
Tham khảo
Theo Angular Grid: Updating Column Definitions
Tải source code về tại đậy