Giới thiệu
Column Definitions chứa cả thuộc tính trạng thái (stateful) và không trạng thái (non-stateful).
Thuộc tính trạng thái (stateful attributes) có thể thay đổi giá trị của chúng theo grid (ví dụ: Sắp xếp cột có thể được thay đổi khi người dùng nhấp vào tiêu đề cột).
Thuộc tính không trạng thái (non-stateful attributes) không thay đổi so với những gì được đặt trong Column Definition (ví dụ: khi Tên tiêu đề được đặt làm một phần của Column Definitions, nó thường không thay đổi).
Danh sách đầy đủ các thuộc tính stateful của columns như sau:
- Width
- Flex
- Pinned
- Sort
- Hide
- AggFunc
- Row Group
- Pivot
- Column Order
Save và apply State
Có hai phương thức API được cung cấp để lấy và thiết lập trạng thái Column. columnApi.getColumnState()
lấy trạng thái cột hiện tại và columnApi.applyColumnState()
đặt trạng thái cột.
// save the columns state const savedState = this.gridColumnApi.getColumnState(); // restore the column state this.gridColumnApi.applyColumnState({ state: savedState });
Xét một ví dụ cụ thể
Ví dụ dưới đây minh họa việc lưu và khôi phục trạng thái cột:
- Nhấp vào ‘Save State’ để lưu Trạng thái Cột.
- Thay đổi một số trạng thái cột, ví dụ: thay đổi kích thước cột, di chuyển cột xung quanh, áp dụng sắp xếp cột hoặc nhóm hàng, v.v.
- Nhấp vào ‘Restore State’ và trạng thái cột được đặt trở lại vị trí ban đầu khi bạn nhấp vào ‘Lưu Trạng thái’.
- Nhấp vào ‘Reset State’ và trạng thái sẽ quay trở lại những gì đã được xác định trong Định nghĩa cột.
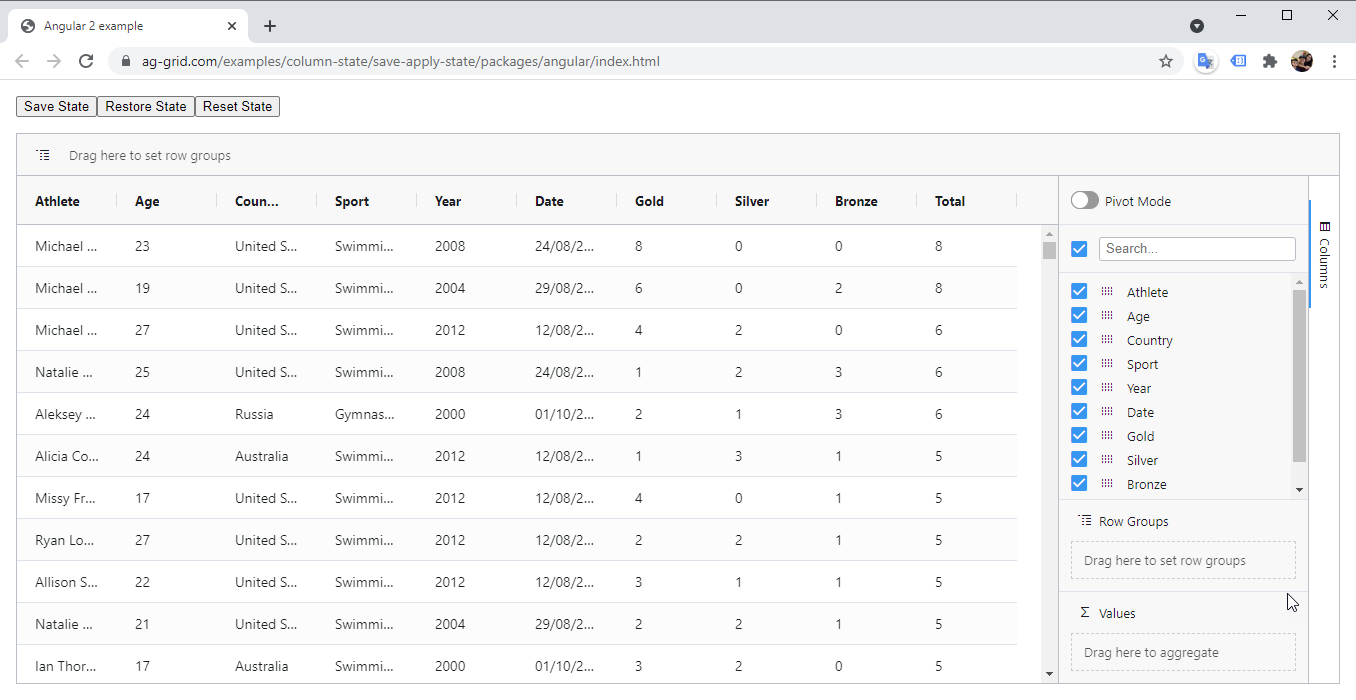
#1. Khởi tạo và cài đặt nhanh grid
Xem hướng dẫn khởi tạo và cài đặt nhanh grid cơ bản cho dự án tại đây
#2. Cài đặt thư viện cần thiết
npm i @ag-grid-enterprise/all-modules npm i @ag-grid-community/angular
#3. Code cho app module
src\app\app.module.ts
import { NgModule } from '@angular/core'; import { BrowserModule } from '@angular/platform-browser'; import {HttpClientModule} from "@angular/common/http"; import { FormsModule } from '@angular/forms'; // ag-grid import { AgGridModule } from '@ag-grid-community/angular'; import { AppComponent } from './app.component'; @NgModule({ declarations: [ AppComponent ], imports: [ BrowserModule, FormsModule, HttpClientModule, AgGridModule.withComponents([]), ], providers: [], bootstrap: [AppComponent] }) export class AppModule { }
#4. Code cho app component
src\app\app.component.ts
import { HttpClient } from '@angular/common/http'; import { Component } from '@angular/core'; import { AllModules } from '@ag-grid-enterprise/all-modules'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.scss'], }) export class AppComponent { //objects dùng truy cập tới grid private gridApi: any; private gridColumnApi: any; //objects cài đặt cho properties của grid modules: any = AllModules; columnDefs: any; defaultColDef: any; sideBar: any; rowGroupPanelShow: any; pivotPanelShow: any; rowData: any; constructor(private http: HttpClient) { //cài đặt column definitions this.columnDefs = [ { field: 'athlete' }, { field: 'age' }, { field: 'country' }, { field: 'sport' }, { field: 'year' }, { field: 'date' }, { field: 'gold' }, { field: 'silver' }, { field: 'bronze' }, { field: 'total' }, ]; //cài đặt column definitions mặc định cho các columns this.defaultColDef = { sortable: true, resizable: true, width: 100, enableRowGroup: true, enablePivot: true, enableValue: true, }; //cài đặt sideBar this.sideBar = { toolPanels: ['columns'] }; //cài đặt rowGroupPanelShow this.rowGroupPanelShow = 'always'; //cài đặt pivotPanelShow this.pivotPanelShow = 'always'; } //xử lý sự kiện "save state" saveState() { (window as any).colState = this.gridColumnApi.getColumnState(); console.log('column state saved'); } //xử lý sự kiện "restore state" restoreState() { if (!(window as any).colState) { console.log('no columns state to restore by, you must save state first'); return; } this.gridColumnApi.applyColumnState({ state: (window as any).colState, applyOrder: true, }); console.log('column state restored'); } //xử lý sự kiện "reset state" resetState() { this.gridColumnApi.resetColumnState(); console.log('column state reset'); } //xử lý sự kiện ngay sau khi grid được khởi tạo onGridReady(params: any) { this.gridApi = params.api; this.gridColumnApi = params.columnApi; //tải dữ liệu hiện thị lên grid this.http .get('https://www.ag-grid.com/example-assets/olympic-winners.json') .subscribe((data) => { this.rowData = data; }); } }
#5. Dữ liệu grid hiện thị
Dưới đây là một phần dữ liệu được hiện thị trên grid, để xem đầy đủ xem tại đây
[ { "athlete": "Michael Phelps", "age": 23, "country": "United States", "year": 2008, "date": "24/08/2008", "sport": "Swimming", "gold": 8, "silver": 0, "bronze": 0, "total": 8 }, { "athlete": "Michael Phelps", "age": 19, "country": "United States", "year": 2004, "date": "29/08/2004", "sport": "Swimming", "gold": 6, "silver": 0, "bronze": 2, "total": 8 }, ]
#6. Code cho app template
src\app\app.component.html
<div class="test-container"> <div class="test-header"> <div class="example-section"> <button (click)="saveState()">Save State</button> <button (click)="restoreState()">Restore State</button> <button (click)="resetState()">Reset State</button> </div> </div> <ag-grid-angular #agGrid style="width: 800px; height: 500px;" id="myGrid" class="ag-theme-balham" [modules]="modules" [columnDefs]="columnDefs" [defaultColDef]="defaultColDef" [sideBar]="sideBar" [rowGroupPanelShow]="rowGroupPanelShow" [pivotPanelShow]="pivotPanelShow" [debug]="true" [rowData]="rowData" (gridReady)="onGridReady($event)" ></ag-grid-angular> </div>
#7. Kết quả
Tải lại trang, ta được kết quả như mong đợi… Thế là xong!
Tham khảo
Theo Angular Grid: Column State
Tải source code về tại đây